Delphi Programming / Object Pascal

[掲載 2009年06月07日] [更新 2013年11月28日] ワード操作フォームEx |
ワード操作フォームEx 参考コード類
編集コマンドとコマンドID |
動作確認等 |
Windows 7 U64(SP1) + Delphi XE Pro + Word 2010 |
コマンド ID ( CommandBars ID ) による操作 |
ワードを含め,Office 製品のメニューとコマンドバーの各ボタン,リボンコントロールの各コントロールには,一連のコマンドID と呼ばれる番号が割当ててあります.これを使用した例です.
ワードの操作には,
- FindControl の引数にコマンド ID を指定して Execute メソッドを実行
- ExecuteMso メソッドの引数にコントロールID 指定して実行
- Dialog オブジェクトの各ダイアログのプロパティを設定して,Execute メソッドを実行
の方法があります.コマンド ID とコントロール ID 値のリストは,以下のページで入手できます.これらの値を使用すると,バージョンに関係なくコマンドを実行できます.もちろん,その機能をサポートしていないバージョンでは例外が発生します. |
フォームにボタンを配置して,以下のコードを書けば,これらの機能を持たせることができます.
ただし,ワードでは,例えば,文字列が選択されていないと [コピー] ボタンは使用不可となっています.この状態を実現するのはちょっと面倒です.トグル動作のなら比較的簡単です.
比較用に,一部のコマンド実行に,フォームに配置した CheckBox1 がチェックされている時は,Word オブジェクトのメソッドを実行するようにしています.
文書上の右クリックメニューでも書式や段落は設定できますが,ボタンを配置して操作するようにしたい場合には利用できるでしょう. |
リスト1
コマンドの実行例の参考コード
(順不同) |
//=============================================================================
// コピー
//=============================================================================
procedure TplWordFormEx2.Button1Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.Copy;
exit;
end;
pType := msoControlButton;
pID := 19;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 切り取り
//=============================================================================
procedure TplWordFormEx2.Button2Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.Cut;
exit;
end;
pType := msoControlButton;
pID := 21;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 貼り付け
//=============================================================================
procedure TplWordFormEx2.Button3Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.Paste;
exit;
end;
pType := msoControlButton;
pID := 22;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// やり直し
//=============================================================================
procedure TplWordFormEx2.Button4Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 128;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 行間 1
//=============================================================================
procedure TplWordFormEx2.Button5Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.ParagraphFormat.Space1;
exit;
end;
pType := msoControlButton;
pID := 54;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 行間 1.5
//=============================================================================
procedure TplWordFormEx2.Button6Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.ParagraphFormat.Space15;
exit;
end;
pType := msoControlButton;
pID := 55;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 行間 2
//=============================================================================
procedure TplWordFormEx2.Button7Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
if CheckBox1.Checked then begin
wdfWordApp.Selection.Range.ParagraphFormat.Space2;
exit;
end;
pType := msoControlButton;
pID := 56;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 書式のコピー/貼り付け
// クリックするとカーソルが刷毛の形となる
// これで目的の文字をドラッグする
//=============================================================================
procedure TplWordFormEx2.Button8Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 108;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 太字
//=============================================================================
procedure TplWordFormEx2.Button9Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 113;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 斜体
//=============================================================================
procedure TplWordFormEx2.Button10Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 114;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 右揃え
//=============================================================================
procedure TplWordFormEx2.Button11Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 121;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 中央揃え
//=============================================================================
procedure TplWordFormEx2.Button12Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 122;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 両端揃え
//=============================================================================
procedure TplWordFormEx2.Button13Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 123;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// カーソル位置に日付を挿入
//=============================================================================
procedure TplWordFormEx2.Button14Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 125;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// カーソル位置に時刻を挿入
//=============================================================================
procedure TplWordFormEx2.Button15Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 126;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// レベル上げ
//=============================================================================
procedure TplWordFormEx2.Button16Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 132;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// レベル下げ
//=============================================================================
procedure TplWordFormEx2.Button17Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 133;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 均等割付
//=============================================================================
procedure TplWordFormEx2.Button18Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 2792;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 段落番号
// 1.
// 2.
// とかが行の先頭に挿入される.トグル動作
//=============================================================================
procedure TplWordFormEx2.Button19Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 11;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// テキストボックス作成
// 以下を実行してからワード文書をクリックで作成
//=============================================================================
procedure TplWordFormEx2.Button20Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 139;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 縦書きテキストボックス
// 以下を実行してからワード文書をクリックで作成
//=============================================================================
procedure TplWordFormEx2.Button21Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 318;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// クリップアート
//=============================================================================
procedure TplWordFormEx2.Button22Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := EmptyParam;
pID := 682;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 改ページ カーソル位置へ挿入ダイアログ
//=============================================================================
procedure TplWordFormEx2.Button23Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := EmptyParam;
pID := 766;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 日付と時刻挿入ダイアログ
//=============================================================================
procedure TplWordFormEx2.Button24Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 768;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 段落設定ダイアログ
//=============================================================================
procedure TplWordFormEx2.Button25Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 779;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 縦書きと横書きダイアログ
//=============================================================================
procedure TplWordFormEx2.Button26Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 782;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 文字カウントダイアログ
//=============================================================================
procedure TplWordFormEx2.Button27Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 792;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 辞書の単語登録ダイアログ
//=============================================================================
procedure TplWordFormEx2.Button28Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 827;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 均等割付 ダイアログ
//=============================================================================
procedure TplWordFormEx2.Button29Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := EmptyParam;
pID := 2792;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// ルビ設定ダイアログ表示
//=============================================================================
procedure TplWordFormEx2.Button30Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 3511;
try
wdfSetFocusWord;
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 文字の網かけ
//=============================================================================
procedure TplWordFormEx2.Button31Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 3518;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 囲み線
//=============================================================================
procedure TplWordFormEx2.Button32Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 3517;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 水平線
//=============================================================================
procedure TplWordFormEx2.Button33Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 3653;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
ボタンをクリックすると,罫線を描画する鉛筆 (ペン),または消しゴムが現れます.これらはトグル表示となっています.
Word 2003 以前の場合,このコマンドを実行すると,ワード操作フォームEx では非表示にしていますが,[罫線] のコマンドバーが表示されます.したがって,ワードを起動した時にこのコマンドバーが表示されることになります. |
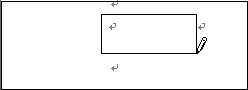 |
図1
罫線ツール |
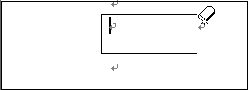 |
図2
消しゴムツール |
//=============================================================================
// 罫線の鉛筆 トグル
//=============================================================================
procedure TplWordFormEx2.Button1Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 2059;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
//=============================================================================
// 罫線の消しゴム トグル
//=============================================================================
procedure TplWordFormEx2.Button2Click(Sender: TObject);
var
pType : OleVariant;
pID : OleVariant;
begin
pType := msoControlButton;
pID := 2060;
try
wdfWordApp.CommandBars.FindControl(pType, pID, EmptyParam, EmptyParam).Execute;
except
raise;
end;
wdfSetFocusWord;
end;
ネット上にはワードを操作するサンプルがありますが,その多くは,VBA で書かれています.
これを Delphi で使用するには,例えば次のように,ワードのインスタンスを OleVarint 型に変換して使用すると,多くの VBA のコードが利用できます.下のコードは,バージョンダイアログを表示する例です. |
リスト3
ワードのオブジェクトのインスタンスを OleVariant 型にして,VBA のコードを実行 |
var
objWordApp : OleVariant;
begin
wdfSetFocusWord;
objWordApp := wdfWordApp.Application;
try
objWordApp.CommandBars.FindControl(ID:=927).Execute;
except
end;
wdfSetFocusWord;
end;
|